(Zepto||jQuery)3行代码开启前端组件复用之旅(一)
Nov 7, 2017ZeptojQuery工具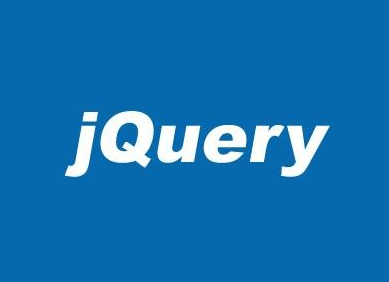
应用场景
日常开发中,会经常遇到一些重复性很强的内容,比方说活动页中的规则、协议弹窗,样式差不多的产品展示等等。这种情况下,可以将这部分常用部分抽离开出来通过按需请求实现多页面共用,提升了开发效率。
可作为组件的特点:
- 重复性:作为组件首先满足的特点肯定是重复性,如果这个组件做出来只可能在一个页面中使用,其意义也不大。可能被多个页面或一个页面多次使用的内容肯定是优先考虑作成组件的。
- 关联性:组件可以跟主页有一定的关联,主要体现在css跟js的共用上,这点是组件跟iframe的主要区别之一。组件跟页面之间可以互相使用样式和方法,实现互利互惠。
- 有一定“份量”: 这里的“份量”指的是代码量、节点数、逻辑复杂度。假设组件只有几句”Hello world!”,那也没意义,并且反而会因为增加请求量弊大于利;相反如果组件过于复杂,那么请求时间也会造成不良的用户体验。所以作为组件,有足够“份量”才能倍有面子。
- 非”首屏”显示:组件是需要请求的,所以为了首屏更快得显示,页面加载进去时的内容最好不要通过请求异步加载。
一.开启——三行代码实现组件复用
1.首先我们先随意写个主页面,index.html
1 | <section class="m-container f-tc"> |
其中“j-renderCtn”是我们将要渲染组件的地方。
2.我们再写个组件,components/test.html
1 | <style> |
组件里写了组件的样式、节点、事件。
3.实现组件加载,三行代码来了,index.html:
1 | <script type="text/javascript"> |
效果图:
(渲染前):
(渲染后):
(点击按钮事件):
通过ajax请求获取组件内的代码放到事先准备的容器内。看起来是不是很low,low吗?确实low。
demo地址:demo
目前的组件开发可吐槽的点太多了。好,那咱就改进改进。。。
二.发展——灵活性,自定义填充内容
现在的组件有一个很严重的问题,文字内容什么都是写死的,那么很尴尬的,差不多的组件如果只是文案或颜色有所不同现在这情况只能再添加个组件。这样的组件太弱了我不要,所以首先就来实现内容的灵活性。
思路:正则匹配,变量替换。咱ajax请求回来的数据不就是字符串么,字符串就好办了嘛
1.变量替换
在组件中,我们以”{ {“开头,”} }“结尾,中间为变量的形式定义的内容,可以被定义变量所替换,若取不到变量则用空字符串替换内容1
{{变量名}}
组件,components/test.html:
1 | <style> |
这里有btnColor,name,content三个变量。
主页面:
1 | <script type="text/javascript"> |
实现效果:
(渲染后):
(点击按钮事件):
可以看到css、html、js里的变量都成功被替换了
demo地址:demo
2.列表渲染
当组件是一个表格或是一个包含标签显示的产品时,列表数量跟标签数量都是不确定的,因此我们还要加个循环
循环以
1 | {{r-for="数组变量"}} |
语法形式编写,当数组变量Arr的各项内容为对象时,变量位置替换为相应的属性(如Arr[index].name),否则变量位置替换为该项数组(如Arr[index])。
组件——components/testtable.html:
1 | ... |
主页面,为了方便这里干脆写了个jQuery函数:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51/*
* @param {Object} options: 组件参数
* {String} options.url: 组件路径;
* {object} options.value: 数据内容;
*/
$.fn.renderComponent = function (options) {
if (!options || !options.url) return false;
var $t = $(this),
_value = options.value;
var reg = /{{(.*)}}/gi,
listReg = /{{r-for="(\w*)"}}/gi;
// 渲染列表获取html
var getListHtml = function (key, html) { // key列表数组变量,html原html
var _reg = new RegExp('{{r-for="' + key + '"}}([\\s\\S*]+?){{r-end}}');
return html.replace(_reg, function (matches, modulehtml) {
var _resultHtml = '';
for (var i = 0; i < _value[key].length; i++) {
_resultHtml += modulehtml.replace(reg, function (_matches, arritem) {
arritem = arritem.trim();
return (typeof _value[key][i] == 'object' ? _value[key][i][arritem] : _value[key][i]) || ''
});
}
return _resultHtml;
});
};
// 请求组件
$.get(options.url, function (htmldata) {
var _listKeys = [];
// 替换列表内容
htmldata.replace(lsitReg, function (matches, m1) {
_listKeys.push(m1);
});
for (var i in _listKeys) {
htmldata = getListHtml(_listKeys[i], htmldata);
}
// 替换其他变量
htmldata = htmldata.replace(reg, function (matches, m1) {
var _m1 = m1.trim();
return _value[_m1] || '';
});
$t.html(htmldata);
});
};
调用:
1 | $('.j-renderCtn').renderComponent({ |
实现效果:
(渲染后):
至此组件有了变量跟列表渲染,瞬间感觉灵活了。
本篇内容初步介绍了前端组件化的开发模式,当前的组件函数具备了变量及循环的功能,但其还存在着很多缺陷,例如一个页面多次引用组件会浪费请求,组件中的css、js可能会影响整个页面等等。下篇将为其进行改善,添加其本地缓存等功能。
谢谢~
Author
My name is Micheal Wayne and this is my blog.
I am a front-end software engineer.
Contact: michealwayne@163.com